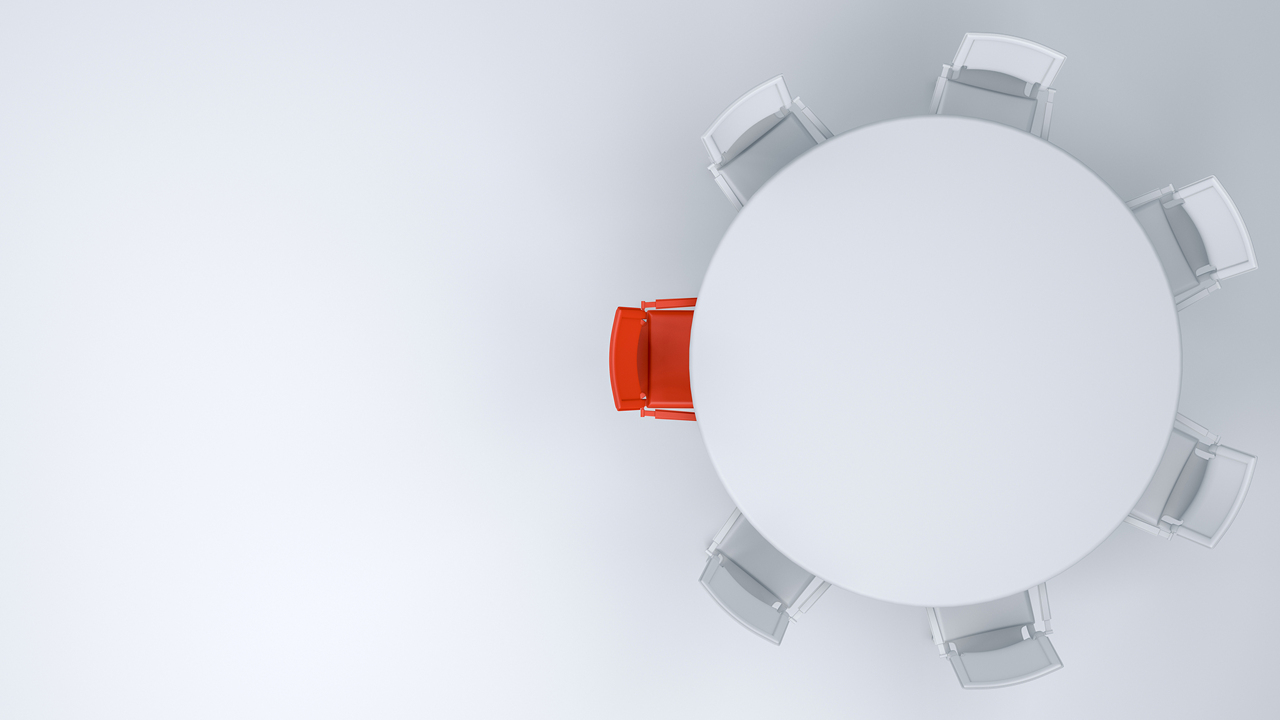
5.2 Formatting
5.2.1 Post Processing
By default, Tplyr will build your table and store it as a tibble. It can be accessed directly in your environment by simply calling it.
head(my_table, n = 9)
## # A tibble: 9 × 9
## row_label1 row_label2 var1_Placebo var1_Total `var1_Xanomeli…`
## <chr> <chr> <chr> <chr> <chr>
## 1 Age (years) N "86" "254" "84"
## 2 Age (years) Mean [SD] "75.21 [ 8.590]" "75.09 [ 8.2… "74.38 [ 7.886]"
## 3 Age (years) Median "76.0" "77.0" "76.0"
## 4 Age (years) Min, Max "52, 89" "51, 89" "56, 88"
## 5 Age Group 1 (years) <65 " 14 [16.28%]" " 33 [12.99%… " 11 [13.10%]"
## 6 Age Group 1 (years) >80 " 30 [34.88%]" " 77 [30.31%… " 18 [21.43%]"
## 7 Age Group 1 (years) 65-80 " 42 [48.84%]" "144 [56.69%… " 55 [65.48%]"
## 8 Gender F " 53 [61.63%]" "143 [56.30%… " 40 [47.62%]"
## 9 Gender M " 33 [38.37%]" "111 [43.70%… " 44 [52.38%]"
## # … with 4 more variables: `var1_Xanomeline Low Dose` <chr>,
## # ord_layer_index <int>, ord_layer_1 <int>, ord_layer_2 <dbl>
Since it is tibble, you are free to further process or wrangle it to your liking. Below we’ll apply some light formatting using a combination of Tplyr
and dplyr
:
- Remove repeating variable labels and insert breaks between variable rows
- Reorder the columns
- Remove extraneous columns not of interest for display (i.e. meta data created by Tplyr)
library(dplyr)
my_table %>%
my_table <-
# remove repeating labels
apply_row_masks(., row_breaks = TRUE) %>%
# specify order of relevant variables
select(row_label1,
row_label2,`var1_Xanomeline High Dose`,
`var1_Xanomeline Low Dose`,
var1_Placebo, var1_Total)
We can see the formatting has taken effect by recalling it in our environment. Specifically, compare the row_label1
variable below to the previous section while also noting the order of columns has changed to our specification.
head(my_table, n = 9)
## # A tibble: 9 × 6
## row_label1 row_label2 `var1_Xanomeli…` `var1_Xanomeli…` var1_Placebo
## <chr> <chr> <chr> <chr> <chr>
## 1 "Age (years)" "N" "84" "84" "86"
## 2 "" "Mean [SD… "74.38 [ 7.886]" "75.67 [ 8.286]" "75.21 [ 8.…
## 3 "" "Median" "76.0" "77.5" "76.0"
## 4 "" "Min, Max" "56, 88" "51, 88" "52, 89"
## 5 "" "" "" "" ""
## 6 "Age Group 1 (years… "<65" " 11 [13.10%]" " 8 [ 9.52%]" " 14 [16.28…
## 7 "" ">80" " 18 [21.43%]" " 29 [34.52%]" " 30 [34.88…
## 8 "" "65-80" " 55 [65.48%]" " 47 [55.95%]" " 42 [48.84…
## 9 "" "" "" "" ""
## # … with 1 more variable: var1_Total <chr>
5.2.2 Displaying
While Tplyr
aided us in building a summary tables, other packages exist to help us display them. One package we will focus on is the flextable
package. This table display package is quite powerful and offers a high degree of customization.
We turn the interested reader to the flextable book to view additional examples.
library(flextable)
# a basic flextable
my_table %>%
my_flextable <-
# start flextable
flextable() %>%
autofit()
my_flextable
row_label1 | row_label2 | var1_Xanomeline High Dose | var1_Xanomeline Low Dose | var1_Placebo | var1_Total |
Age (years) | N | 84 | 84 | 86 | 254 |
Mean [SD] | 74.38 [ 7.886] | 75.67 [ 8.286] | 75.21 [ 8.590] | 75.09 [ 8.246] | |
Median | 76.0 | 77.5 | 76.0 | 77.0 | |
Min, Max | 56, 88 | 51, 88 | 52, 89 | 51, 89 | |
Age Group 1 (years) | <65 | 11 [13.10%] | 8 [ 9.52%] | 14 [16.28%] | 33 [12.99%] |
>80 | 18 [21.43%] | 29 [34.52%] | 30 [34.88%] | 77 [30.31%] | |
65-80 | 55 [65.48%] | 47 [55.95%] | 42 [48.84%] | 144 [56.69%] | |
Gender | F | 40 [47.62%] | 50 [59.52%] | 53 [61.63%] | 143 [56.30%] |
M | 44 [52.38%] | 34 [40.48%] | 33 [38.37%] | 111 [43.70%] | |
Ethnicity | HISPANIC OR LATINO | 3 [ 3.57%] | 6 [ 7.14%] | 3 [ 3.49%] | 12 [ 4.72%] |
NOT HISPANIC OR LATINO | 81 [96.43%] | 78 [92.86%] | 83 [96.51%] | 242 [95.28%] | |
Baseline Body Mass Index (kg/m2) | N | 84 | 84 | 86 | 254 |
Mean [SD] | 25.35 [ 4.158] | 25.06 [ 4.271] | 23.64 [ 3.672] | 24.67 [ 4.092] | |
Median | 24.8 | 24.3 | 23.4 | 24.2 | |
Min, Max | 14, 34 | 18, 40 | 15, 33 | 14, 40 | |
We can apply some flextable
formatting to alter the display of the table.
# a nicer flextable
my_table %>%
my_flextable <-
# start flextable
flextable() %>%
autofit() %>%
# add some padding between rows
padding(padding = 0.5) %>%
# adjust width of first two columns
width(j = 1:2, width = 4) %>%
# align treatment columns to center
align(part = "all", align = "center", j = 3:6) %>%
# column header labels
set_header_labels(., values = list(
row_label1 = 'Variable',
row_label2 = ' ',
`var1_Xanomeline High Dose` = 'Xanomeline \nHigh Dose',
`var1_Xanomeline Low Dose` = 'Xanomeline \nLow Dose',
var1_Placebo = 'Placebo',
var1_Total = 'Total')) %>%
# header + footers
add_header_lines(values = "Table: Demographics (Safety Analysis Set)") %>%
add_footer_lines(values = "This was produced in R!") %>%
# font size, font name
fontsize(part = "all", size = 8)
# font()
# font(part = "all", fontname = "Times")
# This errors, perhaps version issue. -- SZ
Table: Demographics (Safety Analysis Set) | |||||
Variable |
| Xanomeline | Xanomeline | Placebo | Total |
Age (years) | N | 84 | 84 | 86 | 254 |
Mean [SD] | 74.38 [ 7.886] | 75.67 [ 8.286] | 75.21 [ 8.590] | 75.09 [ 8.246] | |
Median | 76.0 | 77.5 | 76.0 | 77.0 | |
Min, Max | 56, 88 | 51, 88 | 52, 89 | 51, 89 | |
Age Group 1 (years) | <65 | 11 [13.10%] | 8 [ 9.52%] | 14 [16.28%] | 33 [12.99%] |
>80 | 18 [21.43%] | 29 [34.52%] | 30 [34.88%] | 77 [30.31%] | |
65-80 | 55 [65.48%] | 47 [55.95%] | 42 [48.84%] | 144 [56.69%] | |
Gender | F | 40 [47.62%] | 50 [59.52%] | 53 [61.63%] | 143 [56.30%] |
M | 44 [52.38%] | 34 [40.48%] | 33 [38.37%] | 111 [43.70%] | |
Ethnicity | HISPANIC OR LATINO | 3 [ 3.57%] | 6 [ 7.14%] | 3 [ 3.49%] | 12 [ 4.72%] |
NOT HISPANIC OR LATINO | 81 [96.43%] | 78 [92.86%] | 83 [96.51%] | 242 [95.28%] | |
Baseline Body Mass Index (kg/m2) | N | 84 | 84 | 86 | 254 |
Mean [SD] | 25.35 [ 4.158] | 25.06 [ 4.271] | 23.64 [ 3.672] | 24.67 [ 4.092] | |
Median | 24.8 | 24.3 | 23.4 | 24.2 | |
Min, Max | 14, 34 | 18, 40 | 15, 33 | 14, 40 | |
This was produced in R! |